Understanding Variables and Functions
- ML Studios
- Jan 31
- 3 min read

Cooking and programming both involve measuring, organizing, and transforming ingredients (data) into a final product (output).
A great way to understand this is by chopping an onion. Before cutting, we have a whole onion, but once chopped, it turns into smaller pieces.
The number of pieces depends on:
The size of the onion (bigger onions yield more pieces).
The chopping technique (finer cuts = more pieces).
This relates to how variables store and transfer information in programming.
Variables: Onion Size vs. Chopped Pieces
A variable is like a storage container for information. In this example:
The size of the onion determines how many pieces we get.
Once chopped, the onion turns into smaller pieces, which we also track using a variable.
Types of Variables in Programming
Programming uses different data types to store different kinds of values.
Data Type | Example in Cooking | What It Stores? |
int (integer) | Number of chopped onion pieces (e.g., 32 pieces) | Whole numbers |
double (floating-point number) | Onion size (e.g., 0.6 for a smaller onion) | Numbers with decimals |
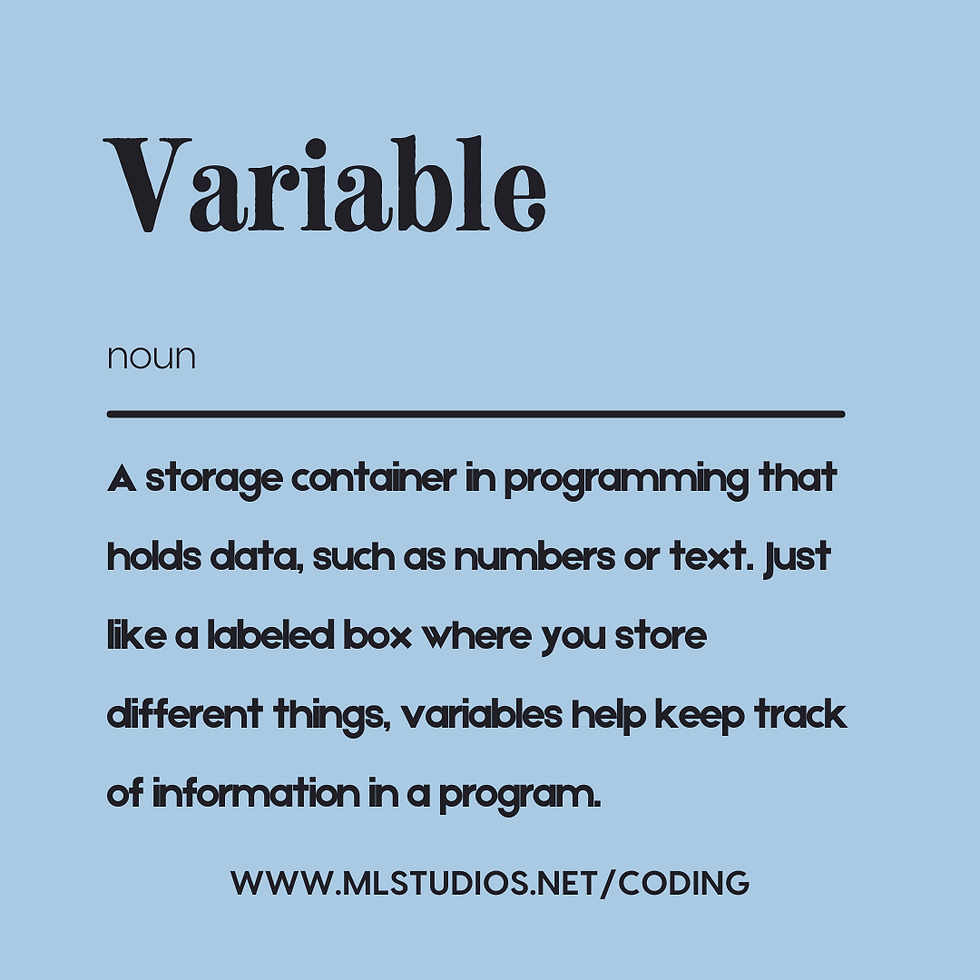
Example in C#
double onionSize = 0.6; // 60% of a normal onion
int pieces = 0; // Starts with 0 pieces before chopping
double stores fractions like an onion’s size (0.6 for 60% normal size).
int stores whole numbers like chopped pieces (32 pieces).
Functions: Chopping an Onion (Processing Data)
A function is a set of instructions that perform a specific task.
When chopping an onion, the number of pieces depends on:
The onion’s size (bigger onions produce more pieces).
A fixed chopping pattern (e.g., 64 pieces per full onion).
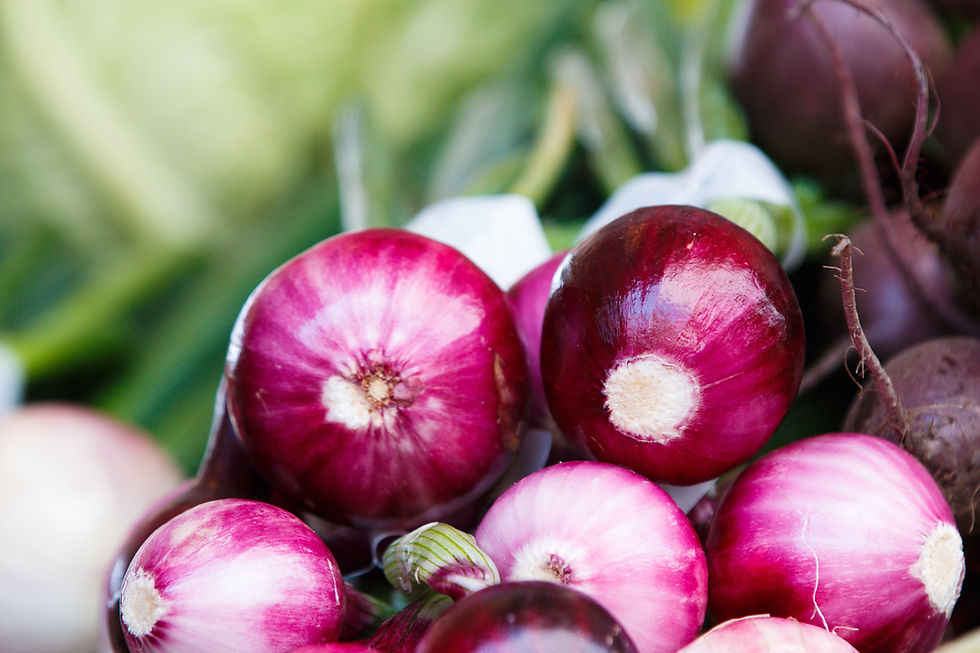
Chopping an Onion (Function Example)
We assume a standard onion produces 64 pieces. If the onion is 60% of normal size, we adjust accordingly:
int ChopOnion(double onionSize)
{
int basePiecesPerOnion = 64; // Standard pieces per full onion
return (int)(onionSize * basePiecesPerOnion);
}
Breaking Down the Code
int ChopOnion(...) → Defines a function that returns an integer (whole number of pieces).
double onionSize → A decimal value that adjusts how many pieces are created.
int basePiecesPerOnion = 64; → We assume a standard onion creates 64 pieces when chopped.
return (int)(onionSize * basePiecesPerOnion); →
Multiply by onionSize (e.g., 0.6 for a smaller onion).
Convert the result to an integer (pieces must be a whole number).
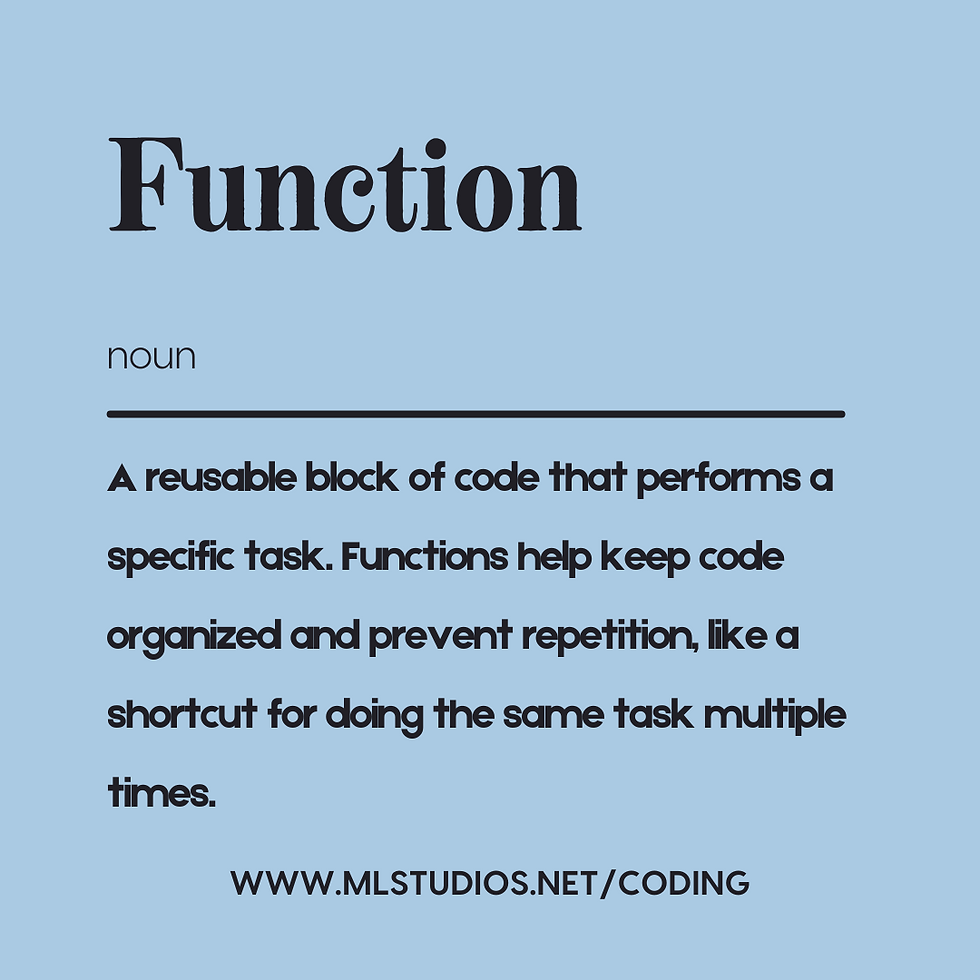
Using the Function in a Program
Now let’s use this function to chop onions of different sizes.
class Program
{
static void Main()
{
double onionSize = 0.6; // 60% of normal onion size
int pieces = ChopOnion(onionSize); // Calculate pieces
Console.WriteLine($"A {onionSize * 100}% sized onion was chopped into {pieces} pieces.");
}
static int ChopOnion(double onionSize)
{
int basePiecesPerOnion = 64; // Standard pieces per full onion
return (int)(onionSize * basePiecesPerOnion);
}
}
Expected Output:
A 60% sized onion was chopped into 38 pieces.
(Since 0.6 * 64 = 38.4, and integers round down to 38).
Tracking Used vs. Remaining Pieces
Once an onion is chopped, we may use some of the pieces in a recipe.
Example:
We chop a 70% onion (producing 45 pieces).
We use 20 pieces in a recipe.
We store the remaining 25 pieces for later.
int totalPieces = ChopOnion(0.7); // Chop a 70% sized onion
int usedPieces = 20; // Amount used for a recipe
int remainingPieces = totalPieces - usedPieces; // What's left
Console.WriteLine($"We used {usedPieces} pieces.");
Console.WriteLine($"Remaining onion pieces: {remainingPieces}");
Expected Output:
We used 20 pieces.
Remaining onion pieces: 25

Conclusion
Variables store ingredient details (double for onion size, int for chopped pieces).
Variable types define measurement units (int for whole numbers, double for decimals).
Functions process and modify data, just like chopping changes an onion into pieces.
Data is transferred between variables, just like tracking ingredients in a recipe.
By thinking of programming as following a recipe, learning variables, functions, and data transfer becomes much easier!
Want to Learn More?
🔥 Get personalized programming tutoring at mlstudios.net/coding and sharpen your coding skills today!
Comments