Organizing Data with Structures and Classes
- ML Studios
- Jan 31
- 3 min read
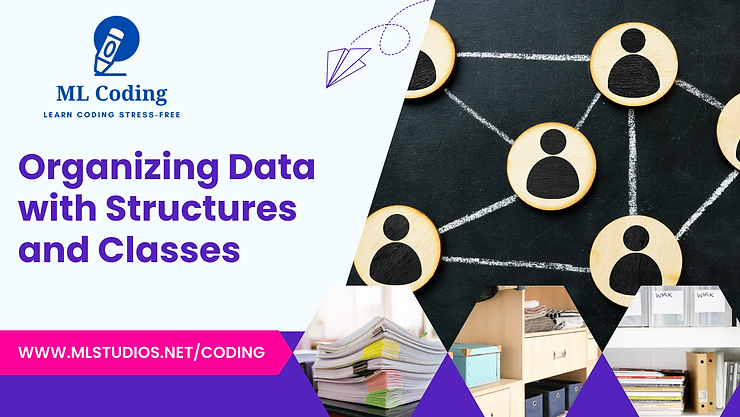
So far, we’ve used variables to track an onion’s size and how many pieces it produces when chopped. While this works for simple tasks, real-world programming often requires handling multiple related details together.
For example, in a kitchen, a chef doesn't just think about one ingredient at a time—they manage multiple ingredients with different properties. Instead of listing separate variables for each ingredient, it’s better to group them into a structured format that represents real-life objects.
This is where structures (struct) and classes (class) come in—they allow us to organize and predefine data in a structured way.
Simplified Representations in Programming
When defining a structure or class, we only include the details that matter for our task.
For example, an onion’s color doesn’t affect chopping, so we don’t need to store that information. Instead, we only track:
Size (affects how many pieces we get).
Chopped state (whether it has been processed or not).
Number of chopped pieces (if applicable).
Using a struct to Organize an Onion’s Data
A struct (structure) is a lightweight way to store related variables inside a single unit.
Defining the Onion Structure
struct Onion
{
public double Size; // Onion size as a percentage (1.0 = normal, 0.6 = smaller onion)
public bool IsChopped; // True if the onion has been chopped
public int Pieces; // Number of pieces after chopping
}
This groups all onion-related data together, so we don’t need separate variables for each detail.
Creating and Using an Onion Struct
Onion myOnion = new Onion();
myOnion.Size = 0.6; // 60% of normal size
myOnion.IsChopped = false;
myOnion.Pieces = 0;
Console.WriteLine($"Onion Size: {myOnion.Size * 100}%");
Console.WriteLine($"Is Chopped: {myOnion.IsChopped}");
Console.WriteLine($"Chopped Pieces: {myOnion.Pieces}");
Expected Output:
Onion Size: 60%
Is Chopped: False
Chopped Pieces: 0
This makes the data much easier to read and manage because everything related to the onion is now stored inside one structured object.
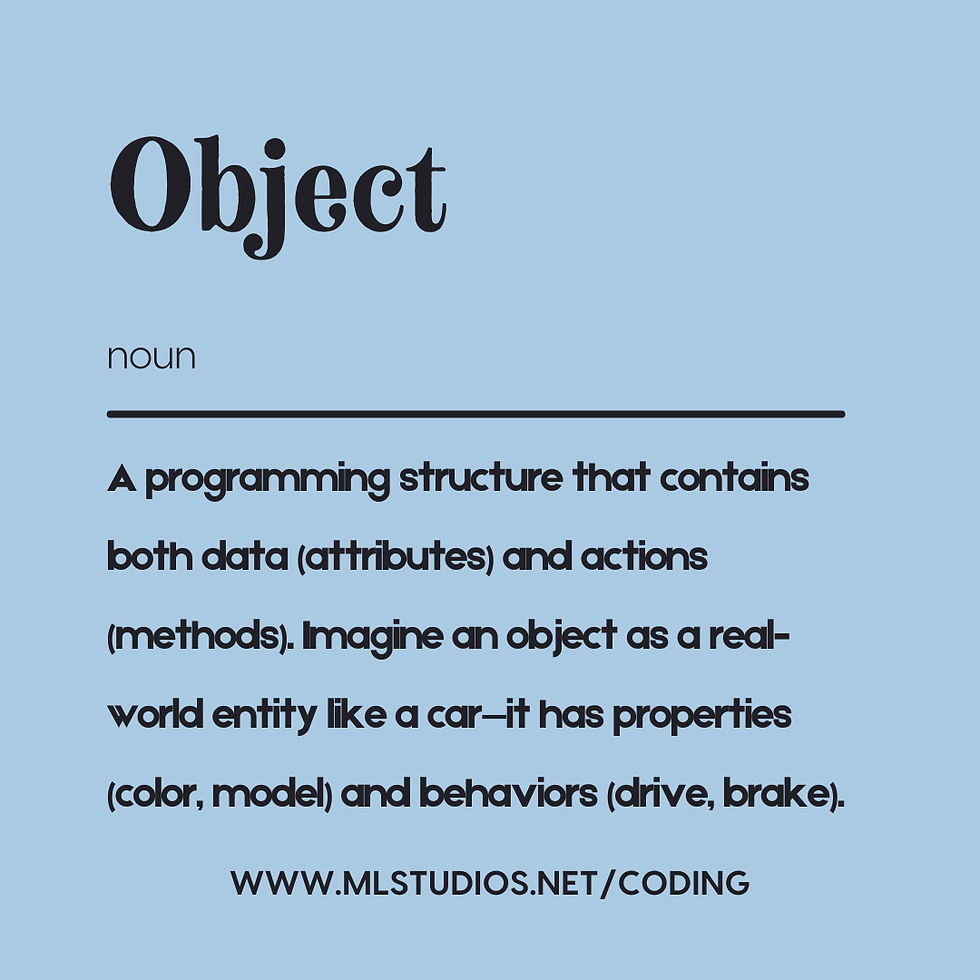
Using a class to Add Functionality
While a struct is great for storing simple data, a class is more powerful because it can store data and perform actions using functions inside the class.
Defining an Onion Class with a Chop Function
class Onion
{
public double Size { get; set; } // Onion size (percentage of normal)
public bool IsChopped { get; private set; } // Track if it's chopped
public int Pieces { get; private set; } // Number of chopped pieces
public Onion(double size)
{
Size = size;
IsChopped = false;
Pieces = 0;
}
public void Chop()
{
if (!IsChopped)
{
int basePieces = 64; // A full onion yields 64 pieces
Pieces = (int)(Size * basePieces); // Adjust by size
IsChopped = true;
Console.WriteLine($"Chopped the onion into {Pieces} pieces.");
}
else
{
Console.WriteLine("This onion is already chopped!");
}
}
}
Breaking Down the Class:
public double Size { get; set; } → Stores onion size.
public bool IsChopped { get; private set; } → Tracks if the onion has already been chopped.
public int Pieces { get; private set; } → Stores how many pieces were created.
public void Chop() → A function inside the class that updates the onion’s chopped state.
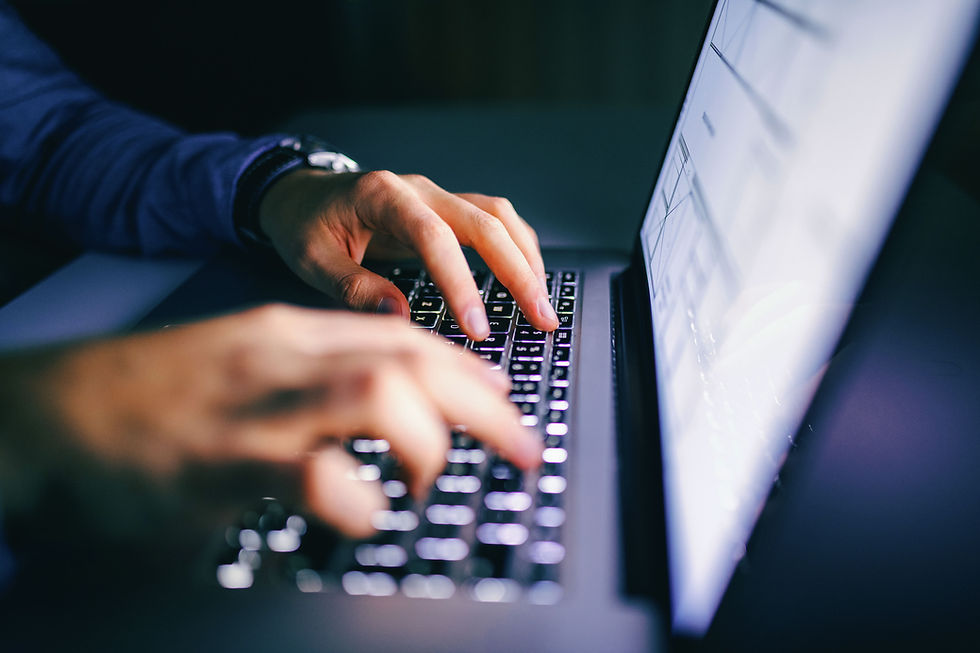
Using the Onion Class in a Program
Now we can create and chop an onion easily:
class Program
{
static void Main()
{
Onion myOnion = new Onion(0.6); // A 60% sized onion
Console.WriteLine($"Onion Size: {myOnion.Size * 100}%");
Console.WriteLine($"Is Chopped: {myOnion.IsChopped}");
myOnion.Chop(); // Chop the onion
Console.WriteLine($"Chopped Pieces: {myOnion.Pieces}");
}
}
Expected Output:
Onion Size: 60%
Is Chopped: False
Chopped the onion into 38 pieces.
Chopped Pieces: 38
(Since 0.6 * 64 = 38.4, and integers round down to 38).
Why Use Classes Instead of Just Functions?
In our previous post, we used separate variables and functions to track onions.
But using a class makes it:
✅ More Organized → All onion-related data is stored together.
✅ Easier to Read → We no longer need separate variables.
✅ Reusable → We can create multiple onions with different sizes without rewriting code.
Key Takeaways
✔ Structures (struct) are lightweight and store related variables together.
✔ Classes (class) can store data AND perform actions using functions.
✔ We only include the details that matter (no need to track onion color if it doesn’t affect chopping).
✔ Using classes makes code cleaner and easier to work with in real-world applications.
By structuring information before writing code, you make programming more efficient and easier to manage—just like how organizing ingredients in a kitchen makes cooking smoother!
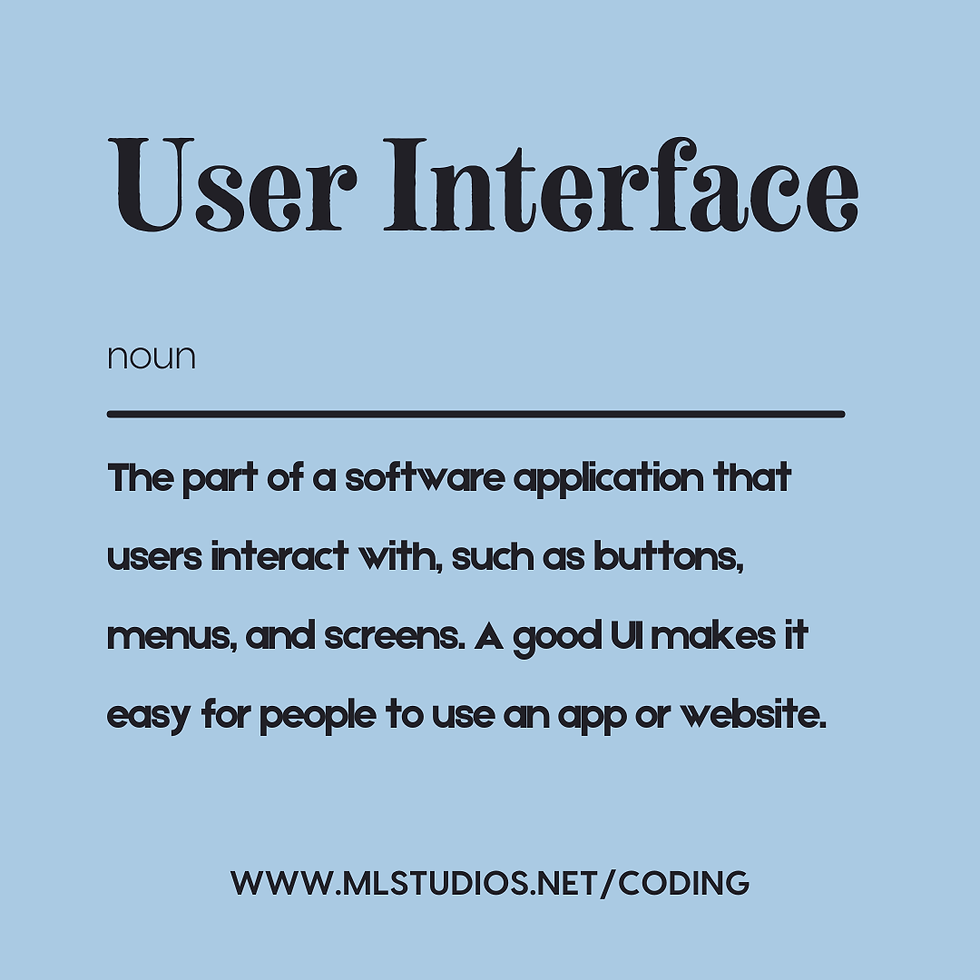
Now you understand how structures (struct) and classes (class) help organize and manage real-life objects in programming.
In our next post, we’ll explore how to handle multiple onions at once using lists and collections—just like managing ingredients for a full recipe!
Want to Learn More?
🔥 Get personalized programming tutoring at mlstudios.net/coding and sharpen your coding skills today!
Comentários